代理式AI的五級式綜合教程:從基礎(chǔ)快速響應(yīng)到全自主代碼生成與執(zhí)行 原創(chuàng)
在本教程中,我們將講解代理式架構(gòu)的五個級別,從最簡單的語言模型調(diào)用到完全自主的代碼生成和執(zhí)行系統(tǒng)。本教程專為在Google Colab上無縫運行而設(shè)計。從一個簡單的“處理器”開始(僅回顯模型輸出),你將逐步構(gòu)建路由邏輯、集成外部工具、編排多步驟工作流,并最終使模型能夠規(guī)劃、驗證、優(yōu)化并執(zhí)行自己的Python代碼。在每個部分中,你都會找到詳細的解釋、自包含的演示函數(shù)以及清晰的提示,展示如何在實際AI應(yīng)用中平衡人工控制與機器自治。
import os
import torch
from transformers import pipeline, AutoTokenizer, AutoModelForCausalLM
import re
import json
import time
import random
from IPython.display import clear_output
我們導(dǎo)入核心Python和第三方庫,包括用于環(huán)境和執(zhí)行控制的os和time,以及Hugging Face的Transformers(pipeline、AutoTokenizer、AutoModelForCausalLM)用于模型加載和推理。此外,我們使用re和json解析LLM輸出、隨機種子和模擬數(shù)據(jù),同時利用clear_output保持整潔的Colab界面。
MODEL_NAME = "TinyLlama/TinyLlama-1.1B-Chat-v1.0"
def get_model_and_tokenizer():
if not hasattr(get_model_and_tokenizer, "model"):
print(f"Loading model {MODEL_NAME}...")
tokenizer = AutoTokenizer.from_pretrained(MODEL_NAME)
model = AutoModelForCausalLM.from_pretrained(
MODEL_NAME,
torch_dtype=torch.float16,
device_map="auto",
low_cpu_mem_usage=True
)
get_model_and_tokenizer.model = model
get_model_and_tokenizer.tokenizer = tokenizer
print("Model loaded successfully!")
return get_model_and_tokenizer.model, get_model_and_tokenizer.tokenizer
我們定義了一個變量MODEL_NAME指向TinyLlama 1.1B聊天模型,并實現(xiàn)了一個懶加載輔助函數(shù)get_model_and_tokenizer(),該函數(shù)僅在首次調(diào)用時下載并初始化分詞器和模型,將其緩存以最小化開銷,并在后續(xù)調(diào)用中返回緩存實例。
def get_model_and_tokenizer():
if not hasattr(get_model_and_tokenizer, "model"):
print(f"Loading model {MODEL_NAME}...")
tokenizer = AutoTokenizer.from_pretrained(MODEL_NAME)
model = AutoModelForCausalLM.from_pretrained(
MODEL_NAME,
torch_dtype=torch.float16,
device_map="auto",
low_cpu_mem_usage=True
)
get_model_and_tokenizer.model = model
get_model_and_tokenizer.tokenizer = tokenizer
print("Model loaded successfully!")
return get_model_and_tokenizer.model, get_model_and_tokenizer.tokenizer
這個輔助函數(shù)為TinyLlama模型及其標記器實現(xiàn)了懶加載模式。在第一次調(diào)用時,它下載并初始化半精度和自動設(shè)備排布,將它們作為函數(shù)對象的屬性緩存,在后續(xù)調(diào)用時,則直接返回已經(jīng)加載的實例以避免冗余開銷。
def generate_text(prompt, max_length=512):
model, tokenizer = get_model_and_tokenizer()
messages = [{"role": "user", "content": prompt}]
formatted_prompt = tokenizer.apply_chat_template(messages, tokenize=False)
inputs = tokenizer(formatted_prompt, return_tensors="pt").to(model.device)
with torch.no_grad():
output = model.generate(
**inputs,
max_new_tokens=max_length,
do_sample=True,
temperature=0.7,
top_p=0.9,
)
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
response = generated_text.split("ASSISTANT: ")[-1].strip()
return response
generate_text函數(shù)封裝了TinyLlama推理流程:它獲取緩存的模型和分詞器,將用戶提示格式化為聊天模板,對輸入進行分詞并移動到模型設(shè)備,然后通過采樣參數(shù)生成響應(yīng)。生成完成后,它解碼輸出并通過“ASSISTANT: ”標記提取助手的回答。
第一級:簡單處理器
在最簡單的級別,代碼定義了一個直接的文本生成管道,將模型純粹視為語言處理器。當用戶提供提示詞時,simple_processor函數(shù)調(diào)用generate_text輔助函數(shù)生成自由形式的響應(yīng),并直接顯示該響應(yīng)。此級別展示了最基本的交互模式:接收輸入、生成輸出,程序流程完全由人工控制。
def simple_processor(prompt):
"""Level 1: Simple Processor - Model has no impact on program flow"""
response = generate_text(prompt)
return response
def demo_level1():
print("\n" + "="*50)
print("LEVEL 1: SIMPLE PROCESSOR DEMO")
print("="*50)
print("At this level, the AI has no control over program flow.")
print("It simply takes input and produces output.\n")
user_input = input("Enter your question or prompt: ") or "Write a short poem about artificial intelligence."
print("\nProcessing your request...\n")
output = simple_processor(user_input)
print("OUTPUT:")
print("-"*50)
print(output)
print("-"*50)
simple_processor函數(shù)體現(xiàn)了智能體層次結(jié)構(gòu)中的簡單處理器,將模型純粹作為文本生成器;它接受用戶提供的提示并委托給generate_text。它返回模型生成的內(nèi)容,無任何分支或決策邏輯。伴隨的demo_level1例程提供了一個最小的交互循環(huán),打印清晰的標題,請求用戶輸入(有合理的默認值),調(diào)用simple_processor,然后顯示原始輸出,展示最基本的提示到響應(yīng)的工作流程,其中AI對程序流程沒有影響。
第二級:路由機制
第二級引入基于模型分類的條件路由。router_agent函數(shù)首先要求模型將查詢分類為“技術(shù)性”、“創(chuàng)造性”或“事實性”,然后規(guī)范化該分類并將其分配到專門的處理函數(shù)(handle_technical_query、handle_creative_query或handle_factual_query)。此路由機制使模型能夠部分控制程序流程,指導(dǎo)后續(xù)交互路徑。
def router_agent(user_query):
"""Level 2: Router - Model determines basic program flow"""
category_prompt = f"""Classify the following query into one of these categories:
'technical', 'creative', or 'factual'.
Query: {user_query}
Return ONLY the category name and nothing else."""
category_response = generate_text(category_prompt)
category = category_response.lower()
if "technical" in category:
category = "technical"
elif "creative" in category:
category = "creative"
else:
category = "factual"
print(f"Query classified as: {category}")
if category == "technical":
return handle_technical_query(user_query)
elif category == "creative":
return handle_creative_query(user_query)
else:
return handle_factual_query(user_query)
def handle_technical_query(query):
system_prompt = f"""You are a technical assistant. Provide detailed technical explanations.
User query: {query}"""
response = generate_text(system_prompt)
return f"[Technical Response]\n{response}"
def handle_creative_query(query):
system_prompt = f"""You are a creative assistant. Be imaginative and inspiring.
User query: {query}"""
response = generate_text(system_prompt)
return f"[Creative Response]\n{response}"
def handle_factual_query(query):
system_prompt = f"""You are a factual assistant. Provide accurate information concisely.
User query: {query}"""
response = generate_text(system_prompt)
return f"[Factual Response]\n{response}"
def demo_level2():
print("\n" + "="*50)
print("LEVEL 2: ROUTER DEMO")
print("="*50)
print("At this level, the AI determines basic program flow.")
print("It decides which processing path to take.\n")
user_query = input("Enter your question or prompt: ") or "How do neural networks work?"
print("\nProcessing your request...\n")
result = router_agent(user_query)
print("OUTPUT:")
print("-"*50)
print(result)
print("-"*50)
router_agent函數(shù)通過首先要求模型將用戶的查詢分類為“技術(shù)性”、“創(chuàng)造性”或“事實性”,然后規(guī)范化該分類并將其分配給相應(yīng)的處理程序(handle_technical_query、handle_creative_query或handle_factual_query),每個處理程序都將原始查詢包裝在適當?shù)南到y(tǒng)風格提示中,然后調(diào)用generate_text來實現(xiàn)路由器行為。demo_level2例程提供了一個清晰的CLI風格界面,打印標題,接受輸入(有默認值),調(diào)用router_agent,并顯示分類后的響應(yīng),展示了模型如何通過對處理路徑的選擇來基本控制程序流程。
第三級:工具調(diào)用
第三級通過嵌入基于JSON的函數(shù)選擇協(xié)議,賦予模型決定調(diào)用哪些外部工具的能力。tool_calling_agent函數(shù)向用戶提供一個問題及一系列潛在工具選項(如天氣查詢、信息搜索、日期時間獲取或直接響應(yīng)),并指示模型返回一個指定工具及其參數(shù)的有效JSON消息。通過正則表達式提取JSON對象后,代碼會安全地回退到直接響應(yīng)以防解析失敗。最后,模型整合工具結(jié)果生成連貫的答案。
def tool_calling_agent(user_query):
"""Level 3: Tool Calling - Model determines how functions are executed"""
tool_selection_prompt = f"""Based on the user query, select the most appropriate tool from the following list:
1. get_weather: Get the current weather for a location
2. search_information: Search for specific information on a topic
3. get_date_time: Get current date and time
4. direct_response: Provide a direct response without using tools
USER QUERY: {user_query}
INSTRUCTIONS:
- Return your response in valid JSON format
- Include the tool name and any required parameters
- For get_weather, include location parameter
- For search_information, include query and depth parameter (basic or detailed)
- For get_date_time, include timezone parameter (optional)
- For direct_response, no parameters needed
Example output format: {{"tool": "get_weather", "parameters": {{"location": "New York"}}}}"""
tool_selection_response = generate_text(tool_selection_prompt)
try:
json_match = re.search(r'({.*})', tool_selection_response, re.DOTALL)
if json_match:
tool_selection = json.loads(json_match.group(1))
else:
print("Could not parse tool selection. Defaulting to direct response.")
tool_selection = {"tool": "direct_response", "parameters": {}}
except json.JSONDecodeError:
print("Invalid JSON in tool selection. Defaulting to direct response.")
tool_selection = {"tool": "direct_response", "parameters": {}}
tool_name = tool_selection.get("tool", "direct_response")
parameters = tool_selection.get("parameters", {})
print(f"Selected tool: {tool_name}")
if tool_name == "get_weather":
location = parameters.get("location", "Unknown")
tool_result = get_weather(location)
elif tool_name == "search_information":
query = parameters.get("query", user_query)
depth = parameters.get("depth", "basic")
tool_result = search_information(query, depth)
elif tool_name == "get_date_time":
timezone = parameters.get("timezone", "UTC")
tool_result = get_date_time(timezone)
else:
return generate_text(f"Please provide a helpful response to: {user_query}")
final_prompt = f"""User Query: {user_query}
Tool Used: {tool_name}
Tool Result: {json.dumps(tool_result)}
Based on the user's query and the tool result above, provide a helpful response."""
final_response = generate_text(final_prompt)
return final_response
def get_weather(location):
weather_conditions = ["Sunny", "Partly cloudy", "Overcast", "Light rain", "Heavy rain", "Thunderstorms", "Snowy", "Foggy"]
temperatures = {
"cold": list(range(-10, 10)),
"mild": list(range(10, 25)),
"hot": list(range(25, 40))
}
location_hash = sum(ord(c) for c in location)
condition_index = location_hash % len(weather_conditions)
season = ["winter", "spring", "summer", "fall"][location_hash % 4]
temp_range = temperatures["cold"] if season in ["winter", "fall"] else temperatures["hot"] if season == "summer" else temperatures["mild"]
temperature = random.choice(temp_range)
return {
"location": location,
"temperature": f"{temperature}°C",
"conditions": weather_conditions[condition_index],
"humidity": f"{random.randint(30, 90)}%"
}
def search_information(query, depth="basic"):
mock_results = [
f"First result about {query}",
f"Second result discussing {query}",
f"Third result analyzing {query}"
]
if depth == "detailed":
mock_results.extend([
f"Fourth detailed analysis of {query}",
f"Fifth comprehensive overview of {query}",
f"Sixth academic paper on {query}"
])
return {
"query": query,
"results": mock_results,
"depth": depth,
"sources": [f"source{i}.com" for i in range(1, len(mock_results) + 1)]
}
def get_date_time(timezone="UTC"):
current_time = time.strftime("%Y-%m-%d %H:%M:%S", time.gmtime())
return {
"current_datetime": current_time,
"timezone": timezone
}
def demo_level3():
print("\n" + "="*50)
print("LEVEL 3: TOOL CALLING DEMO")
print("="*50)
print("At this level, the AI selects which tools to use and with what parameters.")
print("It can process the results from tools to create a final response.\n")
user_query = input("Enter your question or prompt: ") or "What's the weather like in San Francisco?"
print("\nProcessing your request...\n")
result = tool_calling_agent(user_query)
print("OUTPUT:")
print("-"*50)
print(result)
print("-"*50)
在第三級實現(xiàn)中,tool_calling_agent函數(shù)提示模型從預(yù)定義的實用程序集中選擇,例如天氣查詢、模擬網(wǎng)絡(luò)搜索或日期/時間檢索,通過返回一個包含選定工具名稱及其參數(shù)的JSON對象。然后,它安全地解析該JSON,調(diào)用相應(yīng)的Python函數(shù)以獲得結(jié)構(gòu)化數(shù)據(jù),并進行后續(xù)模型調(diào)用,將工具的輸出整合到一個連貫的面向用戶的響應(yīng)中。
第四級:多步智能體
第四級擴展了工具調(diào)用模式,形成一個多步智能體,管理其工作流和狀態(tài)。MultiStepAgent類維護用戶輸入、工具輸出和智能體操作的內(nèi)部記憶。每次迭代生成一個規(guī)劃提示,總結(jié)整個記憶,要求模型選擇幾個工具之一,如網(wǎng)絡(luò)搜索模擬、信息提取、文本摘要或報告創(chuàng)建,或完成任務(wù)并生成最終輸出。執(zhí)行所選工具并將結(jié)果附加回記憶后,重復(fù)該過程,直到模型發(fā)出“完成”動作或達到最大步驟數(shù)。最后,智能體將記憶整理成一個連貫的最終響應(yīng)。這種結(jié)構(gòu)展示了LLM如何協(xié)調(diào)復(fù)雜的多階段過程,同時咨詢外部函數(shù)并根據(jù)先前結(jié)果細化其計劃。
class MultiStepAgent:
"""Level 4: Multi-Step Agent - Model controls iteration and program continuation"""
def __init__(self):
self.tools = {
"search_web": self.search_web,
"extract_info": self.extract_info,
"summarize_text": self.summarize_text,
"create_report": self.create_report
}
self.memory = []
self.max_steps = 5
def run(self, user_task):
self.memory.append({"role": "user", "content": user_task})
steps_taken = 0
while steps_taken < self.max_steps:
next_action = self.determine_next_action()
if next_action["action"] == "complete":
return next_action["output"]
tool_name = next_action["tool"]
tool_args = next_action["args"]
print(f"\n Step {steps_taken + 1}: Using tool '{tool_name}' with arguments: {tool_args}")
tool_result = self.tools[tool_name](**tool_args)
self.memory.append({
"role": "tool",
"content": json.dumps(tool_result)
})
steps_taken += 1
return self.generate_final_response("Maximum steps reached. Here's what I've found so far.")
def determine_next_action(self):
context = "Current memory state:\n"
for item in self.memory:
if item["role"] == "user":
context += f"USER INPUT: {item['content']}\n\n"
elif item["role"] == "tool":
context += f"TOOL RESULT: {item['content']}\n\n"
prompt = f"""{context}
Based on the above information, determine the next action to take.
Choose one of the following options:
1. search_web: Search for information (args: query)
2. extract_info: Extract specific information from a text (args: text, target_info)
3. summarize_text: Create a summary of text (args: text)
4. create_report: Create a structured report (args: title, content)
5. complete: Task is complete (include final output)
Respond with a JSON object with the following structure:
For tools: {{"action": "tool", "tool": "tool_name", "args": {{tool-specific arguments}}}}
For completion: {{"action": "complete", "output": "final output text"}}
Only return the JSON object and nothing else."""
next_action_response = generate_text(prompt)
try:
json_match = re.search(r'({.*})', next_action_response, re.DOTALL)
if json_match:
next_action = json.loads(json_match.group(1))
else:
return {"action": "complete", "output": "I encountered an error in planning. Here's what I know so far: " + self.generate_final_response("Error in planning")}
except json.JSONDecodeError:
return {"action": "complete", "output": "I encountered an error in planning. Here's what I know so far: " + self.generate_final_response("Error in planning")}
self.memory.append({"role": "assistant", "content": next_action_response})
return next_action
def generate_final_response(self, prefix=""):
context = "Task history:\n"
for item in self.memory:
if item["role"] == "user":
context += f"USER INPUT: {item['content']}\n\n"
elif item["role"] == "tool":
context += f"TOOL RESULT: {item['content']}\n\n"
elif item["role"] == "assistant":
context += f"AGENT ACTION: {item['content']}\n\n"
prompt = f"""{context}
{prefix} Generate a comprehensive final response that addresses the original user task."""
final_response = generate_text(prompt)
return final_response
def search_web(self, query):
time.sleep(1)
query_hash = sum(ord(c) for c in query)
num_results = (query_hash % 3) + 2
results = []
for i in range(num_results):
results.append(f"Result {i+1}: Information about '{query}' related to aspect {chr(97 + i)}.")
return {
"query": query,
"results": results
}
def extract_info(self, text, target_info):
time.sleep(0.5)
return {
"extracted_info": f"Extracted information about '{target_info}' from the text: The text indicates that {target_info} is related to several key aspects mentioned in the content.",
"confidence": round(random.uniform(0.7, 0.95), 2)
}
def summarize_text(self, text):
time.sleep(0.5)
word_count = len(text.split())
return {
"summary": f"Summary of the provided text ({word_count} words): The text discusses key points related to the subject matter, highlighting important aspects and providing context.",
"original_length": word_count,
"summary_length": round(word_count * 0.3)
}
def create_report(self, title, content):
time.sleep(0.7)
report_sections = [
"## Introduction",
f"This report provides an overview of {title}.",
"",
"## Key Findings",
content,
"",
"## Conclusion",
f"This analysis of {title} highlights several important aspects that warrant consideration."
]
return {
"report": "\n".join(report_sections),
"word_count": len(content.split()),
"section_count": 3
}
def demo_level4():
print("\n" + "="*50)
print("LEVEL 4: MULTI-STEP AGENT DEMO")
print("="*50)
print("At this level, the AI manages the entire workflow, deciding which tools")
print("to use, when to use them, and determining when the task is complete.\n")
user_task = input("Enter a research or analysis task: ") or "Research quantum computing recent developments and create a brief report"
print("\nProcessing your request... (this may take a minute)\n")
agent = MultiStepAgent()
result = agent.run(user_task)
print("\nFINAL OUTPUT:")
print("-"*50)
print(result)
print("-"*50)
MultiStepAgent類維護用戶輸入和工具輸出的演變記憶,然后反復(fù)提示LLM決定其下一步行動,無論是搜索網(wǎng)絡(luò)、提取信息、匯總文本、創(chuàng)建報告還是完成任務(wù),執(zhí)行所選工具并將結(jié)果附加到記憶中,直到任務(wù)完成或達到步驟上限。在此過程中,它展示了第四級智能體如何通過讓模型控制迭代和程序繼續(xù)來協(xié)調(diào)多步驟工作流。
第五級:完全自主智能體
在最高層次上,AutonomousAgent類展示了一個閉環(huán)系統(tǒng),其中模型不僅負責規(guī)劃和執(zhí)行,還能生成、驗證、優(yōu)化并運行新的Python代碼。記錄用戶任務(wù)后,智能體要求模型生成詳細計劃,然后提示其生成獨立的解決方案代碼,自動清除Markdown格式。隨后的驗證步驟查詢模型是否存在語法或邏輯問題;如果發(fā)現(xiàn)問題,則要求模型優(yōu)化代碼。驗證后的代碼被包裝了沙箱實用程序,例如安全打印、捕獲輸出緩沖區(qū)和結(jié)果捕獲邏輯,并在受限制的本地環(huán)境中執(zhí)行。最后,智能體合成了一份專業(yè)報告,解釋了所做的工作、完成方式和最終結(jié)果。這一級別展示了真正自主的AI系統(tǒng),可以通過動態(tài)代碼創(chuàng)建和執(zhí)行擴展其能力。
class AutonomousAgent:
"""Level 5: Fully Autonomous Agent - Model creates & executes new code"""
def __init__(self):
self.memory = []
def run(self, user_task):
self.memory.append({"role": "user", "content": user_task})
print(" Planning solution approach...")
planning_message = self.plan_solution(user_task)
self.memory.append({"role": "assistant", "content": planning_message})
print(" Generating solution code...")
generated_code = self.generate_solution_code()
self.memory.append({"role": "assistant", "content": f"Generated code: ```python\n{generated_code}\n```"})
print(" Validating code...")
validation_result = self.validate_code(generated_code)
if not validation_result["valid"]:
print(" Code validation found issues - refining...")
refined_code = self.refine_code(generated_code, validation_result["issues"])
self.memory.append({"role": "assistant", "content": f"Refined code: ```python\n{refined_code}\n```"})
generated_code = refined_code
else:
print(" Code validation passed")
try:
print(" Executing solution...")
execution_result = self.safe_execute_code(generated_code, user_task)
self.memory.append({"role": "system", "content": f"Execution result: {execution_result}"})
# Generate a final report
print(" Creating final report...")
final_report = self.create_final_report(execution_result)
return final_report
except Exception as e:
return f"Error executing the solution: {str(e)}\n\nGenerated code was:\n```python\n{generated_code}\n```"
def plan_solution(self, task):
prompt = f"""Task: {task}
You are an autonomous problem-solving agent. Create a detailed plan to solve this task.
Include:
1. Breaking down the task into subtasks
2. What algorithms or approaches you'll use
3. What data structures are needed
4. Any external resources or libraries required
5. Expected challenges and how to address them
Provide a step-by-step plan.
"""
return generate_text(prompt)
def generate_solution_code(self):
context = "Task and planning information:\n"
for item in self.memory:
if item["role"] == "user":
context += f"USER TASK: {item['content']}\n\n"
elif item["role"] == "assistant":
context += f"PLANNING: {item['content']}\n\n"
prompt = f"""{context}
Generate clean, efficient Python code that solves this task. Include comments to explain the code.
The code should be self-contained and able to run inside a Python script or notebook.
Only include the Python code itself without any markdown formatting.
"""
code = generate_text(prompt)
code = re.sub(r'^```python\n|```$', '', code, flags=re.MULTILINE)
return code
def validate_code(self, code):
prompt = f"""Code to validate:
```python
{code}
```
Examine the code for the following issues:
1. Syntax errors
2. Logic errors
3. Inefficient implementations
4. Security concerns
5. Missing error handling
6. Import statements for unavailable libraries
If the code has any issues, describe them in detail. If the code looks good, state "No issues found."
"""
validation_response = generate_text(prompt)
if "no issues" in validation_response.lower() or "code looks good" in validation_response.lower():
return {"valid": True, "issues": None}
else:
return {"valid": False, "issues": validation_response}
def refine_code(self, original_code, issues):
prompt = f"""Original code:
```python
{original_code}
```
Issues identified:
{issues}
Please provide a corrected version of the code that addresses these issues.
Only include the Python code itself without any markdown formatting.
"""
refined_code = generate_text(prompt)
refined_code = re.sub(r'^```python\n|```$', '', refined_code, flags=re.MULTILINE)
return refined_code
def safe_execute_code(self, code, user_task):
safe_imports = """
# Standard library imports
import math
import random
import re
import time
import json
from datetime import datetime
# Define a function to capture printed output
captured_output = []
original_print = print
def safe_print(*args, **kwargs):
output = " ".join(str(arg) for arg in args)
captured_output.append(output)
original_print(output)
print = safe_print
# Define a result variable to store the final output
result = None
# Function to store the final result
def store_result(value):
global result
result = value
return value
"""
result_capture = """
# Store the final result if not already done
if 'result' not in locals() or result is None:
try:
# Look for variables that might contain the final result
potential_results = [var for var in locals() if not var.startswith('_') and var not in
['math', 'random', 're', 'time', 'json', 'datetime',
'captured_output', 'original_print', 'safe_print',
'result', 'store_result']]
if potential_results:
# Use the last defined variable as the result
store_result(locals()[potential_results[-1]])
except:
pass
"""
full_code = safe_imports + "\n# User code starts here\n" + code + "\n\n" + result_capture
code_lines = code.split('\n')
first_lines = code_lines[:3]
print(f"\nExecuting (first 3 lines):\n{first_lines}")
local_env = {}
try:
exec(full_code, {}, local_env)
return {
"output": local_env.get('captured_output', []),
"result": local_env.get('result', "No explicit result returned")
}
except Exception as e:
return {"error": str(e)}
def create_final_report(self, execution_result):
if isinstance(execution_result.get('output'), list):
output_text = "\n".join(execution_result.get('output', []))
else:
output_text = str(execution_result.get('output', ''))
result_text = str(execution_result.get('result', ''))
error_text = execution_result.get('error', '')
context = "Task history:\n"
for item in self.memory:
if item["role"] == "user":
context += f"USER TASK: {item['content']}\n\n"
prompt = f"""{context}
EXECUTION OUTPUT:
{output_text}
EXECUTION RESULT:
{result_text}
{f"ERROR: {error_text}" if error_text else ""}
Create a final report that explains the solution to the original task. Include:
1. What was done
2. How it was accomplished
3. The final results
4. Any insights or conclusions drawn from the analysis
Format the report in a professional, easy to read manner.
"""
return generate_text(prompt)
def demo_level5():
print("\n" + "="*50)
print("LEVEL 5: FULLY AUTONOMOUS AGENT DEMO")
print("="*50)
print("At this level, the AI generates and executes code to solve complex problems.")
print("It can create, validate, refine, and run custom code solutions.\n")
user_task = input("Enter a data analysis or computational task: ") or "Analyze a dataset of numbers [10, 45, 65, 23, 76, 12, 89, 32, 50] and create visualizations of the distribution"
print("\nProcessing your request... (this may take a minute or two)\n")
agent = AutonomousAgent()
result = agent.run(user_task)
print("\nFINAL REPORT:")
print("-"*50)
print(result)
print("-"*50)
AutonomousAgent類體現(xiàn)了完全自主智能體的自主性,包括維護用戶任務(wù)的連續(xù)記憶,并系統(tǒng)協(xié)調(diào)五大核心階段:規(guī)劃、代碼生成、驗證、安全執(zhí)行和報告。啟動運行時,智能體會提示模型生成解決任務(wù)的詳細計劃,并將該計劃存儲在記憶中。接下來,它要求模型基于該計劃創(chuàng)建獨立的Python代碼,去掉任何Markdown格式,并通過查詢模型語法、邏輯、性能和安全問題來驗證代碼。如果驗證發(fā)現(xiàn)任何問題,智能體還會指示模型優(yōu)化代碼,直至通過檢查。最終代碼被包裝在一個沙箱執(zhí)行框架中,包含捕獲的輸出緩沖區(qū)和自動結(jié)果提取,并在隔離的本地環(huán)境中執(zhí)行。最后,智能體通過將執(zhí)行結(jié)果反饋給模型,生成一份精心制作的專業(yè)報告,具體解釋做了什么、如何完成以及獲得了哪些見解。隨附的demo_level5函數(shù)則提供一個簡單的交互循環(huán),即接受用戶任務(wù)、運行智能體并呈現(xiàn)全面的最終報告。
Main函數(shù):囊括以上各步驟
def main():
while True:
clear_output(wait=True)
print("\n" + "="*50)
print("AI AGENT LEVELS DEMO")
print("="*50)
print("\nThis notebook demonstrates the 5 levels of AI agents:")
print("1. Simple Processor - Model has no impact on program flow")
print("2. Router - Model determines basic program flow")
print("3. Tool Calling - Model determines how functions are executed")
print("4. Multi-Step Agent - Model controls iteration and program continuation")
print("5. Fully Autonomous Agent - Model creates & executes new code")
print("6. Quit")
choice = input("\nSelect a level to demo (1-6): ")
if choice == "1":
demo_level1()
elif choice == "2":
demo_level2()
elif choice == "3":
demo_level3()
elif choice == "4":
demo_level4()
elif choice == "5":
demo_level5()
elif choice == "6":
print("\nThank you for exploring the AI Agent levels!")
break
else:
print("\nInvalid choice. Please select 1-6.")
input("\nPress Enter to return to the main menu...")
if __name__ == "__main__":
main()
最后,main函數(shù)會呈現(xiàn)一個簡單的交互菜單循環(huán),清除Colab輸出以提高可讀性,顯示所有五個智能體級別以及退出選項,然后將用戶的選擇分發(fā)到相應(yīng)的演示函數(shù),再等待輸入返回到菜單。這種結(jié)構(gòu)提供了一個集中的CLI風格界面,使你可以按順序探索每個智能體級別,而無需手動介入各具體環(huán)節(jié)。
通過這五個級別的實踐,我們深入了解了代理式AI的原則以及控制、靈活性和自治之間的權(quán)衡。我們看到系統(tǒng)如何從簡單的提示詞響應(yīng)行為演變?yōu)閺?fù)雜的決策管道,甚至自我修改代碼執(zhí)行。無論你是希望開發(fā)智能助手、構(gòu)建數(shù)據(jù)管道還是試驗新興AI能力,這一漸進框架都為設(shè)計強大且可擴展的智能體提供了堅實的路線指導(dǎo)。
原文標題:??A Comprehensive Tutorial on the Five Levels of Agentic AI Architectures: From Basic Prompt Responses to Fully Autonomous Code Generation and Execution?,作者:Asif Razzaq
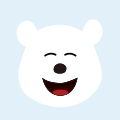